if (timePassed){
do thing
}
Another common stumbling block for less experienced coders, is timing events.
Whether its blinking an LED, or updating a screen with a new sensor value, generally we need some time to pass in between switching an LED state, or a sensor value being updated, else we will not see the LED blinking 8 million times a second, or the sensor value will be changing so quickly we will not be able to read it!
Usually the first tool employed to perform this task for is delay()
delay(1000);
Delay works by hanging the program on this line until the number of milliseconds we pass as an argument has elapsed. In that time the microcontroller is unable to do anything else, read sensors, detect a button press or listen for incoming data.
This method may be perfectly useable if your project is doing a single task, and does not require user intervention, however if we want to code complex behaviours, it is generally going to be advantageous to find a way around this.
Lets have a look at some code that might use delay();
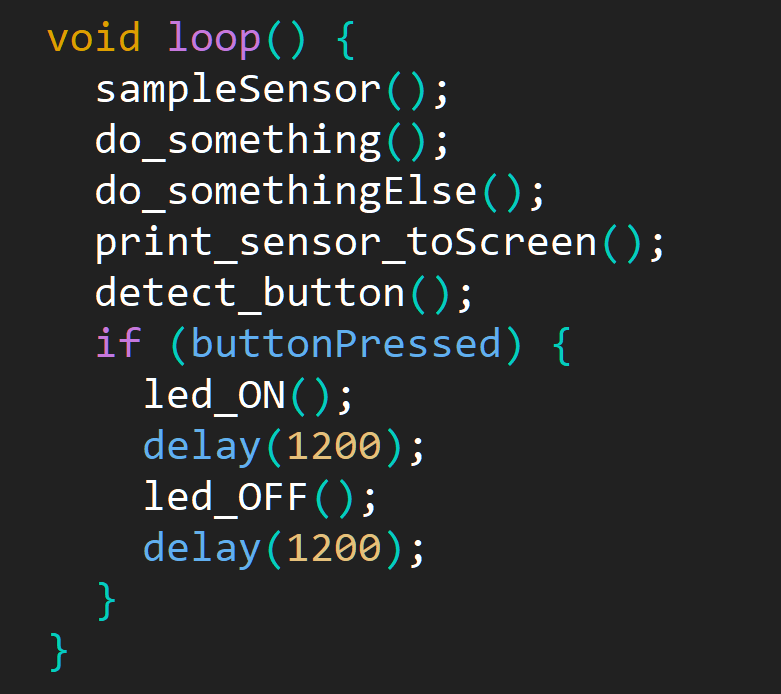
In this situation, once the button press is detected, the program will turn on the LED, then hang for over a second, turn it off, then hang for another second. There is certainly a better way we could do this!
Welcome to the mills(); function.
millis(); returns the total milliseconds that have elapsed since the controller has booted up. To work out how much time has passed between two events, all we need is to record the millis(); value at the time of the first event, then subtract this from the millis(); value at the time of the 2nd event.
To make this work, we will use a global variable to record the value returned from millis() at the time of the first event.

We will also create a variable to hold our desired delay time between our two timed events. In this example we are aiming for a 1 second delay, which is 1000 milliseconds.

In our main loop, we use an if statement, to check if:
The time in milliseconds that has passed since we turned the controller on, minus the time saved when the last event happened,
is greater than or equal to our predetermined event_delayTime.

It’s that simple! It works out only slightly more complex than using delay(). Okay, it uses 2 extra variables, but outside of that its 3 lines of code!
If we implement this code as we have in the example above within a main loop, this is going to repeat the behaviour inside the if() statements {curly brackets}, every event_delayTime milliseconds.
The rest of the time our microcontroller will be running through its other functions, giving us space to do lots of other fun things during runtime.
But what if we only want to delay something once? Well there are many different ways we could do this. Personally I find the easiest is setting boolean flags.
Using Booleans as Control Flags
- Coming Soon